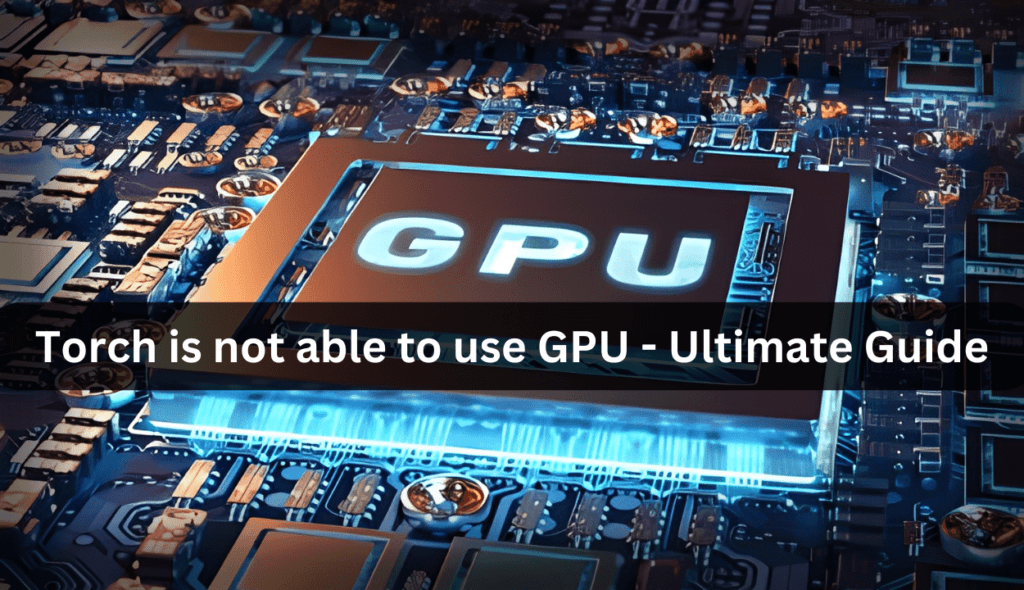
Encountering the frustrating “Torch is not able to use GPU” error can significantly slow down your PyTorch projects. But fear not, fellow developers! This quick fix guide will equip you with the crucial troubleshooting steps to unlock the power of your GPU and accelerate your deep learning workflows.
Why Torch May Not Detect Your GPU?
Have you installed PyTorch on your computer with an NVIDIA GPU, but Torch isn’t using it? There are a few common reasons why Torch may not detect your GPU. Let’s go through some possible solutions to get your GPU working with Torch.
Outdated GPU Drivers
The first thing to check is if your NVIDIA GPU drivers are up to date. Outdated drivers can prevent Torch from detecting your GPU. Go to NVIDIA’s website, download the latest drivers for your GPU model and operating system, and install them.
CUDA Toolkit Mismatch
Torch requires the CUDA toolkit to utilize NVIDIA GPUs. Make sure you have CUDA 10.2 or above installed, which matches the version Torch was compiled with. You can check your CUDA version in the NVIDIA control panel. If it’s outdated, download and install the latest CUDA toolkit.
Incorrect Build Installation
When you installed PyTorch, did you install the correct build? You need to install the build with CUDA and GPU support. For example, on Linux install the pip install torchvision command. On Windows, install the build with CUDA and GPU in the PyTorch installer. Installing the wrong build is a common mistake that prevents Torch from detecting your GPU.
Permissions Issues
If you’re on Linux or macOS, there may be permissions issues preventing Torch from accessing your GPU. Try running torch commands with sudo to give admin permissions. If that fixes the issue, you may need to adjust permissions so your user account can access the GPU. Consult documentation for your OS to resolve permissions problems.
Checking these possible issues and solutions should get your GPU working with Torch in no time.Torch and GPUs can sometimes require troubleshooting, but with patience you’ll get it working.
Testing GPU Availability in Torch

So you’ve installed PyTorch and are ready to get started building neural networks, but your code won’t run on the GPU. Frustrating, right? Don’t worry, there are a few common reasons this may happen and some easy fixes.
Check Your PyTorch Installation
Ensure you installed the GPU-compatible PyTorch and torchvision (matching CUDA version). If not, uninstall and reinstall the correct versions.
Update Your NVIDIA Drivers
Update your graphics card driver from NVIDIA’s website, then reboot to potentially fix PyTorch GPU access issues.
Set the CUDA Environment Variable
If you’re still getting a ‘CUDA error’ when running your PyTorch code, you may need to set the CUDA environment variable. In your terminal/command prompt, enter:
export CUDA_VISIBLE_DEVICES=0
Replace 0 with the ID of your NVIDIA GPU (you can check this in the NVIDIA Control Panel). This tells PyTorch to use that specific GPU.
Check Your Code
Finally, double check that your code is actually trying to run operations on the GPU. You need to set the device to cuda():
device = torch.device("cuda" if torch.cuda.is_available() else "cpu") model.to(device)
This will send your model to the GPU if one is detected, otherwise it will default to the CPU.With any luck, one of these steps should get your PyTorch code up and running on the GPU.
Common Errors When Using GPU in Torch
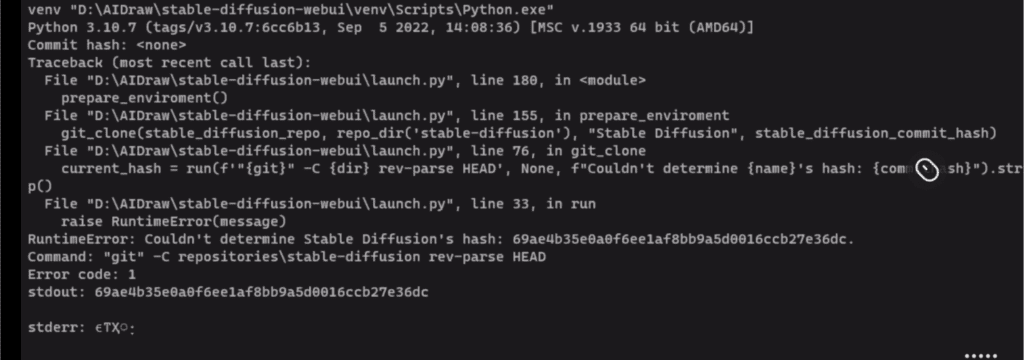
1. Forgetting to Install CUDA
The biggest mistake people make is forgetting to install NVIDIA’s CUDA toolkit. CUDA is required to use your NVIDIA graphics card for computing purposes. Head over to NVIDIA’s website and download the latest version of CUDA for your operating system and graphics card.
2. Not Installing the Correct Version of PyTorch
After installing CUDA, you need to install the GPU version of PyTorch. On the PyTorch website, make sure you install the version that corresponds to your CUDA version. Installing the CPU version of PyTorch will not allow you to utilize your GPU.
3. Forgetting to Move Your Model to the GPU
Even with CUDA and the GPU version of PyTorch installed, your model will still run on the CPU by default. You need to explicitly move your model to the GPU. You can do this by calling .cuda()
on your model. For example, if your model is named model, you would run:
model = model.cuda()
This will move your model to the first GPU (GPU 0) on your system. To move to a different GPU, pass the device index, e.g. model = model.cuda(1)
would move to the second GPU.
4. Not Moving Your Inputs and Labels to the GPU
In addition to moving your model to the GPU, you need to move your inputs and labels to the GPU as well. If you have a batch of inputs inputs and labels labels, run:
inputs = inputs.cuda()
labels = labels.cuda()
This will move your data to the same GPU as your model. If you skip this step, PyTorch will automatically move the data back and forth between the CPU and GPU, which is very inefficient!
Avoiding these common mistakes will get your PyTorch model training on your NVIDIA GPU in no time!
Fixing “Torch Is Not Able to Use GPU”
It’s frustrating when you get the error “Torch is not able to use GPU” when trying to leverage the power of your graphics card for deep learning. But don’t worry, there are a few common reasons this happens and ways to fix it.
Update or Reinstall CUDA
- CUDA is NVIDIA’s parallel computing platform that allows Torch to utilize your GPU. Make sure you have the latest version of CUDA installed that is compatible with your graphics card. You may need to uninstall the current version and do a fresh install of the latest release.
Install the Correct Version of PyTorch
- PyTorch needs to be built specifically for your version of CUDA. Double check that you installed the variant of PyTorch that matches your CUDA version. For example, if you have CUDA 10.1, install PyTorch 1.4 which supports CUDA 10.1. Reinstalling the correct version of PyTorch often resolves the “Torch is not able to use GPU” error.
Choose the Right Build of Torchvision
- Similar to PyTorch, you need to install the build of Torchvision that is compatible with your CUDA and PyTorch versions. Reinstalling the proper version of Torchvision will allow Torch to detect your GPU.
Check Your GPU Drivers
- Outdated or improperly installed GPU drivers are a common reason for the “Torch is not able to use GPU” error. Update your NVIDIA graphics drivers to the latest release to ensure maximum compatibility with CUDA and PyTorch. You may need to uninstall your current drivers before installing the update.
Double Check GPU Settings
- Finally, open your NVIDIA control panel and verify that “CUDA – GPUs” is selected under “CUDA – General”. This will allow Torch to see your GPU. You should also check under “Manage 3D Settings” that your GPU is selected as the preferred processor for “CUDA – GPUs “. Performing these checks will troubleshoot any system setting issues preventing Torch from utilizing your GPU.
With CUDA, PyTorch, Torchvision, GPU drivers, and system settings all properly installed and configured, Torch should now be able to detect and use your GPU for accelerated deep learning.
Also Read: PyTorch Check If GPU Is Available? Quick Guide
Efficient PyTorch GPU Usage Tips
Description | |
Leverage DataLoaders for Efficient Data Movement | Employing PyTorch’s DataLoader helps streamline data loading to the GPU, minimizing bottlenecks and maximizing utilization. |
Embrace the Power of torch.cuda.amp for Mixed Precision | Activate torch.cuda.amp to train models using mixed precision (FP16) on compatible GPUs. This reduces memory usage for significant speed boosts. |
Batch Size Optimization | Experiment with different batch sizes to strike a balance between GPU memory limitations and computational efficiency. Larger batches might not always be best. |
Utilize Model Parallelization for Large Models | For extremely large models exceeding GPU memory capacity, explore model parallelization techniques to distribute training across multiple GPUs. |
Monitor and Profile: Identify Bottlenecks | Use built-in tools like torch.autograd.profiler to pinpoint performance bottlenecks in your code. This allows you to focus optimization efforts effectively. |
Explore frameworks | Consider exploring frameworks like Hugging Face Transformers for state-of-the-art model implementations often pre-optimized for efficient GPU usage. |
FAQs
What is Torch and why is it not utilizing my GPU?
- Torch is a popular machine learning library known for its flexibility and ease of use. If it’s not utilizing your GPU, it could be due to various reasons such as incorrect installation, driver issues, or misconfiguration.
How can I check if Torch is using my GPU?
- You can verify if Torch is utilizing your GPU by running simple commands or scripts provided by Torch. If it’s not, there might be underlying issues that need troubleshooting.
What are the common reasons for Torch not utilizing the GPU?
- Common reasons include outdated GPU drivers, incompatible versions of Torch or CUDA, improper configuration of environment variables, or hardware issues.
How to solve “Torch is not able to use GPU”error?
- You can start by ensuring your GPU drivers are up to date, verifying Torch and CUDA compatibility, setting correct environment variables, and troubleshooting any hardware-related problems.
Does Torch support GPU acceleration?
- Yes, Torch supports GPU acceleration through CUDA. However, it requires proper setup and configuration to utilize the GPU effectively.
I followed the installation instructions, but Torch still doesn’t use my GPU. What should I do?
- Double-check the installation steps, ensuring you followed them correctly. If the issue persists, try reinstalling Torch and CUDA, making sure they are compatible versions.
Are there any specific configurations needed to enable GPU usage in Torch?
- Yes, you may need to set environment variables such as CUDA_HOME and PATH to point to the correct directories containing CUDA binaries and libraries.
Can outdated GPU drivers affect Torch’s performance?
- Absolutely. Outdated GPU drivers can prevent Torch from recognizing and utilizing the GPU efficiently. Make sure to update your GPU drivers regularly.
Is there a way to troubleshoot Torch GPU usage issues?
- Yes, you can troubleshoot Torch GPU usage issues by checking system logs, verifying hardware compatibility, and consulting Torch community forums for assistance.
Where can I find additional help with Torch GPU utilization problems?
- You can seek help from online communities, forums, or official documentation for Torch. Additionally, consider reaching out to experts or consulting with peers who have experience with Torch and GPU utilization.
Why Torch can’t use GPU but it could before?
- Outdated software (PyTorch, CUDA, drivers) or hardware changes can cause Torch to lose GPU access. Update software, check hardware configuration, and consult online resources for further troubleshooting.
Conclusion
- So there you have it – a few quick fixes to try when Torch is not able to use GPU. First, double check your drivers are up to date and compatible. If that doesn’t work, try reinstalling Torch or upgrading to the latest version. Sometimes it can be as simple as enabling CUDA in your environment variables. And as a last resort, you may need to switch the GPU you’re using or install fresh drivers.
- If you encounter further issues, don’t hesitate to consult the official PyTorch documentation and vibrant online communities for expert guidance. With a little troubleshooting, you can harness the power of your GPU and streamline your deep learning workflows with Torch!
Other Articles
- What is GPU Cache? Comprehensive Guide – 2024
- GPU Fans Not Spinning? Here’s How to Fix It
- Do GPU Fans Always Spin? Answering the Big Question
- What is GPU Hotspot Temperature? The Ultimate Guide